Core Data
Core Data is an object-graph management and persistence framework
Core Data stack
A Core Data stack is composed of the following objects: one or more managed object contexts connected to a single persistent store coordinator which is in turn connected to one or more persistent stores. A stack contains all the Core Data components you need to fetch, create, and manipulate managed objects. Minimally it contains:
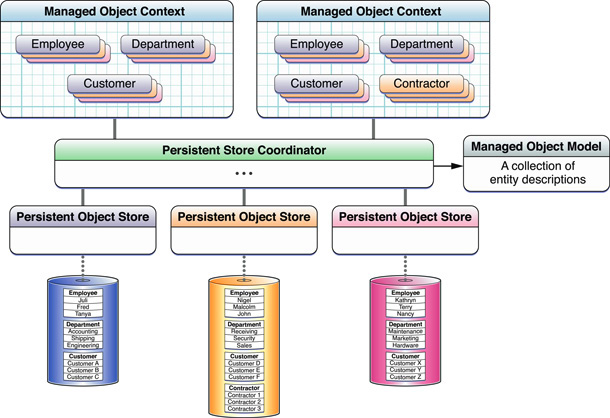
Managed object
A managed object is a model object (in the model-view-controller sense) that represents a record from a persistent store. A managed object is an instance of NSManagedObject or a subclass of NSManagedObject.A managed object has a reference to an entity description object that tells it what entity it represents.
Managed object context
Its primary responsibility is to manage a collection of managed objects. These managed objects represent an internally consistent view of one or more persistent stores.A context is connected to a parent object store. This is usually a persistent store coordinator, but may be another managed object context. When you fetch objects, the context asks its parent object store to return those objects that match the fetch request. Changes that you make to managed objects are not committed to the parent store until you save the context.
Persistent store coordinator
A persistent store coordinator associates persistent object stores and a managed object model, and presents a facade to managed object contexts such that a group of persistent stores appears as a single aggregate store. It has a reference to a managed object model that describes the entities in the store or stores it manages. In complex applications there may be several, each potentially containing different entities. The persistent store coordinator’s role is to manage these stores and present to its managed object contexts the facade of a single unified store. When you fetch records, Core Data retrieves results from all of them, unless you specify which store you’re interested in.
Persistent store
A persistent store is a repository in which managed objects may be stored. You can think of a persistent store as a database data file where individual records each hold the last-saved values of a managed object. Core Data offers three native file types for a persistent store: binary, XML, and SQLite
Mapping model
A Core Data mapping model describes the transformations that are required to migrate data described by a source managed object model to the schema described by a destination model. When you make a new version of a managed object model, you need to migrate persistent stores from the old schema to the new.
Multiple persistent stores
Your app has a fixed data set that is included as part of the bundle Some of the data your app handles is sensitive information that you don’t wish to be persisted on disk You have different storage requirements for different entities
You may have dealt with the first requirement by copying a file from your bundle into a writable location and using it as the base of your entire data store. You may have dealt with the second by manually deleting objects. A separate persistent store is a much better solution in both cases. Each persistent store has its own characteristics - it can be read-only, stored as binary or SQLite or in-memory (on OS X, an XML backing store is also available), or your own implementation of an NSIncrementalStore
one configuration per store each entity should be added to one configuration only.You can’t make relationships between entities in different stores
http://commandshift.co.uk/blog/2013/06/06/multiple-persistent-stores-in-core-data/
Core Data, Multithreading, and the Main Thread
In Core Data, the managed object context can be used with two concurrency patterns, defined by NSMainQueueConcurrencyType and NSPrivateQueueConcurrencyType.
NSMainQueueConcurrencyType is specifically for use with your application interface and can only be used on the main queue of an application
The NSPrivateQueueConcurrencyType configuration creates its own queue upon initialization and can be used only on that queue. Because the queue is private and internal to the NSManagedObjectContext instance, it can only be accessed through the performBlock: and the performBlockAndWait: methods
https://developer.apple.com/library/content/documentation/Cocoa/Conceptual/CoreData/Concurrency.html#//apple_ref/doc/uid/TP40001075-CH24-SW1
Core Data Model Versioning and Data Migration
To migrate a store, you need both the version of the model used to create it, and the current version of the model you want to migrate to.To help Core Data perform the migration, though, you may have to provide information about how to map from one version of the model to another.
Model File Format and Versions
A managed object model that supports versioning is represented in the filesystem by a .xcdatamodeld document. An .xcdatamodeld document is a file package (see Document Packages) that groups versions of the model, each represented by an individual .xcdatamodel file, and an Info.plist file that contains the version information.
The model is compiled into a runtime format—a file package with a .momd extension that contains individually compiled model files with a .mom extension.
https://developer.apple.com/library/content/documentation/Cocoa/Conceptual/CoreDataVersioning/Articles/vmLightweightMigration.html#//apple_ref/doc/uid/TP40004399-CH4-SW1
Subclass of NSManagedObject
What happens if you modify an entity and generate the files for the entity's NSManagedObject subclass again?
Whenever you generate the files for an entity, Xcode will only replace the files of the extension. In other words, if you add an attribute to the Item entity and generate the files for the NSManagedObject subclass, Xcode replaces Item+CoreDataProperties.swift, leaving Item.swift untouched. That's why Xcode tells you to add functionality in Item.swift.
https://code.tutsplus.com/tutorials/core-data-and-swift-subclassing-nsmanagedobject--cms-25116
Core Data Stack Design
In general, avoid doing data processing on the main queue that is not user-related. Data processing can be CPU-intensive, and if it is performed on the main queue, it can result in unresponsiveness in the user interface
NSPersistentContainer
NSPersistentContainer simplifies the creation and management of the Core Data stack by handling the creation of the managed object model (NSManagedObjectModel), persistent store coordinator (NSPersistentStoreCoordinator), and the managed object context (NSManagedObjectContext).
Managed object contexts have a parent store from which they retrieve data representing managed objects and through which they commit changes to managed objects.
the parent store may be another managed object context. Ultimately the root of a context’s ancestry must be a persistent store coordinator.
When you save changes in a context, the changes are only committed “one store up.” If you save a child context, changes are pushed to its parent. Changes are not saved to the persistent store until the root context is saved. (A root managed object context is one whose parent context is nil.) In addition, a parent does not pull changes from children before it saves. You must save a child context if you want ultimately to commit the changes.
private/main both type of ManagedObjectContext can save to persistence Store
https://developer.apple.com/documentation/coredata/nsmanagedobjectcontext#topics
https://www.bignerdranch.com/blog/introducing-the-big-nerd-ranch-core-data-stack/
Fault
Fault handling is transparent—you do not have to execute a fetch to realize a fault. If at some stage a persistent property of a fault object is accessed, Core Data automatically retrieves the data for the object and initializes the object. This process is commonly referred to as firing the fault. If you access a property on the Department object — its name, for example — the fault fires and Core Data executes a fetch for you to retrieve all of the object's attributes.
Core Data automatically fires faults when a persistent property (such as firstName) of a fault is accessed. However, firing faults individually can be inefficient, and there are better strategies for getting data from the persistent store
Uniquing Ensures a Single Managed Object per Record per Context
https://developer.apple.com/library/content/documentation/Cocoa/Conceptual/CoreData/FaultingandUniquing.html
Existing sqlite database
There is no way to directly incorporate into CoreData. It needs to read through 3rd party library and insert in CoreData/ use 3rd party library in overall application to manage that sqlite database.
Core Data stack
A Core Data stack is composed of the following objects: one or more managed object contexts connected to a single persistent store coordinator which is in turn connected to one or more persistent stores. A stack contains all the Core Data components you need to fetch, create, and manipulate managed objects. Minimally it contains:
- An external persistent store that contains saved records.
- A persistent object store that maps between records in the store and objects in your application.
- A persistent store coordinator that aggregates all the stores.
- A managed object model that describes the entities in the stores.
- A managed object context that provides a scratch pad for managed objects.
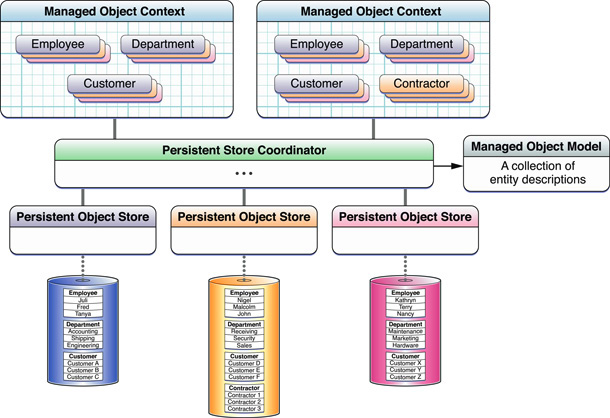
Managed object
A managed object is a model object (in the model-view-controller sense) that represents a record from a persistent store. A managed object is an instance of NSManagedObject or a subclass of NSManagedObject.A managed object has a reference to an entity description object that tells it what entity it represents.
Managed object context
Its primary responsibility is to manage a collection of managed objects. These managed objects represent an internally consistent view of one or more persistent stores.A context is connected to a parent object store. This is usually a persistent store coordinator, but may be another managed object context. When you fetch objects, the context asks its parent object store to return those objects that match the fetch request. Changes that you make to managed objects are not committed to the parent store until you save the context.
Persistent store coordinator
A persistent store coordinator associates persistent object stores and a managed object model, and presents a facade to managed object contexts such that a group of persistent stores appears as a single aggregate store. It has a reference to a managed object model that describes the entities in the store or stores it manages. In complex applications there may be several, each potentially containing different entities. The persistent store coordinator’s role is to manage these stores and present to its managed object contexts the facade of a single unified store. When you fetch records, Core Data retrieves results from all of them, unless you specify which store you’re interested in.
Persistent store
A persistent store is a repository in which managed objects may be stored. You can think of a persistent store as a database data file where individual records each hold the last-saved values of a managed object. Core Data offers three native file types for a persistent store: binary, XML, and SQLite
Mapping model
A Core Data mapping model describes the transformations that are required to migrate data described by a source managed object model to the schema described by a destination model. When you make a new version of a managed object model, you need to migrate persistent stores from the old schema to the new.
Multiple persistent stores
Your app has a fixed data set that is included as part of the bundle Some of the data your app handles is sensitive information that you don’t wish to be persisted on disk You have different storage requirements for different entities
You may have dealt with the first requirement by copying a file from your bundle into a writable location and using it as the base of your entire data store. You may have dealt with the second by manually deleting objects. A separate persistent store is a much better solution in both cases. Each persistent store has its own characteristics - it can be read-only, stored as binary or SQLite or in-memory (on OS X, an XML backing store is also available), or your own implementation of an NSIncrementalStore
one configuration per store each entity should be added to one configuration only.You can’t make relationships between entities in different stores
http://commandshift.co.uk/blog/2013/06/06/multiple-persistent-stores-in-core-data/
Core Data, Multithreading, and the Main Thread
In Core Data, the managed object context can be used with two concurrency patterns, defined by NSMainQueueConcurrencyType and NSPrivateQueueConcurrencyType.
NSMainQueueConcurrencyType is specifically for use with your application interface and can only be used on the main queue of an application
The NSPrivateQueueConcurrencyType configuration creates its own queue upon initialization and can be used only on that queue. Because the queue is private and internal to the NSManagedObjectContext instance, it can only be accessed through the performBlock: and the performBlockAndWait: methods
https://developer.apple.com/library/content/documentation/Cocoa/Conceptual/CoreData/Concurrency.html#//apple_ref/doc/uid/TP40001075-CH24-SW1
Core Data Model Versioning and Data Migration
To migrate a store, you need both the version of the model used to create it, and the current version of the model you want to migrate to.To help Core Data perform the migration, though, you may have to provide information about how to map from one version of the model to another.
Model File Format and Versions
A managed object model that supports versioning is represented in the filesystem by a .xcdatamodeld document. An .xcdatamodeld document is a file package (see Document Packages) that groups versions of the model, each represented by an individual .xcdatamodel file, and an Info.plist file that contains the version information.
The model is compiled into a runtime format—a file package with a .momd extension that contains individually compiled model files with a .mom extension.
https://developer.apple.com/library/content/documentation/Cocoa/Conceptual/CoreDataVersioning/Articles/vmLightweightMigration.html#//apple_ref/doc/uid/TP40004399-CH4-SW1
Subclass of NSManagedObject
What happens if you modify an entity and generate the files for the entity's NSManagedObject subclass again?
Whenever you generate the files for an entity, Xcode will only replace the files of the extension. In other words, if you add an attribute to the Item entity and generate the files for the NSManagedObject subclass, Xcode replaces Item+CoreDataProperties.swift, leaving Item.swift untouched. That's why Xcode tells you to add functionality in Item.swift.
https://code.tutsplus.com/tutorials/core-data-and-swift-subclassing-nsmanagedobject--cms-25116
Core Data Stack Design
In general, avoid doing data processing on the main queue that is not user-related. Data processing can be CPU-intensive, and if it is performed on the main queue, it can result in unresponsiveness in the user interface
NSPersistentContainer
NSPersistentContainer simplifies the creation and management of the Core Data stack by handling the creation of the managed object model (NSManagedObjectModel), persistent store coordinator (NSPersistentStoreCoordinator), and the managed object context (NSManagedObjectContext).
Managed object contexts have a parent store from which they retrieve data representing managed objects and through which they commit changes to managed objects.
the parent store may be another managed object context. Ultimately the root of a context’s ancestry must be a persistent store coordinator.
When you save changes in a context, the changes are only committed “one store up.” If you save a child context, changes are pushed to its parent. Changes are not saved to the persistent store until the root context is saved. (A root managed object context is one whose parent context is nil.) In addition, a parent does not pull changes from children before it saves. You must save a child context if you want ultimately to commit the changes.
private/main both type of ManagedObjectContext can save to persistence Store
https://developer.apple.com/documentation/coredata/nsmanagedobjectcontext#topics
https://www.bignerdranch.com/blog/introducing-the-big-nerd-ranch-core-data-stack/
Fault
Fault handling is transparent—you do not have to execute a fetch to realize a fault. If at some stage a persistent property of a fault object is accessed, Core Data automatically retrieves the data for the object and initializes the object. This process is commonly referred to as firing the fault. If you access a property on the Department object — its name, for example — the fault fires and Core Data executes a fetch for you to retrieve all of the object's attributes.
Core Data automatically fires faults when a persistent property (such as firstName) of a fault is accessed. However, firing faults individually can be inefficient, and there are better strategies for getting data from the persistent store
Uniquing Ensures a Single Managed Object per Record per Context
https://developer.apple.com/library/content/documentation/Cocoa/Conceptual/CoreData/FaultingandUniquing.html
Existing sqlite database
There is no way to directly incorporate into CoreData. It needs to read through 3rd party library and insert in CoreData/ use 3rd party library in overall application to manage that sqlite database.
Comments
Post a Comment